I started by sketching an idea for a system where colorful balls drop at a steady interval, each one representing a moment in time. As more balls fall, it visually shows the passage of timeāthe more balls, the more time has passed.
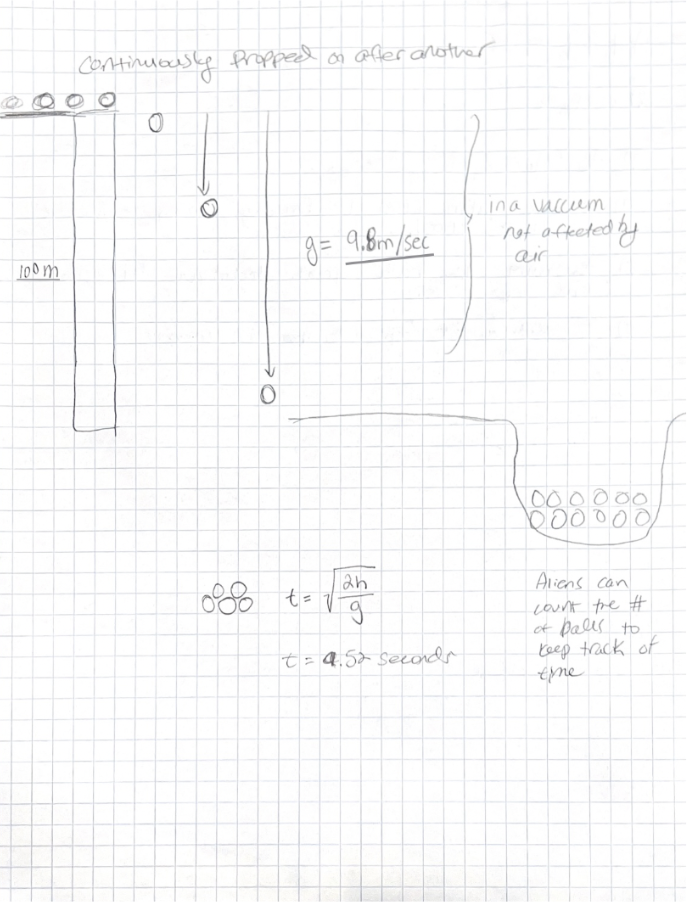
Create an animation that shows a clock for aliens. The clock cannot display time tied to the rotation of the Earth - years, months, days, hours, minutes, etc. Instead create your own system of time - however large or small. If the clock is no longer tied to celestial movement - how else can you express the passing of time? Would the time be continuous or discrete? Cyclical or unidirectional? How would you divide it into sub-elements and what visual tools would you use to represent distinctions: color, shape, size, order? Use arrays and loops. Use rotation with the transformation matrix. Use either trigonometric functions or intervals (or both if need be).
When you set out to develop a reliable method for telling time, one of the critical challenges is finding something that behaves in a consistent, predictable, and repeatable manner. At first glance, there are many possible candidates. A natural thought could be to consider processes like half-lives, where substances decay over time, or the steady drip of water into a bucket. These are both appealing because they offer a form of "counting" or progression that might lend itself to time measurement. However, after further reflection, gravity emerges as an ideal choice.
I started by sketching an idea for a system where colorful balls drop at a steady interval, each one representing a moment in time. As more balls fall, it visually shows the passage of timeāthe more balls, the more time has passed.
This code simulates balls falling from the top of the screen and bouncing off the ground using gravity. The gravity variable is set to 0.5, and it affects how fast the balls fall. The code creates balls that appear in random colors and fall in quick succession, with new balls spawning every second. The for
loop is used to update the position and speed of each ball, checking if it hits the ground and making it bounce with a reduced speed. I used for
loops to handle repeating tasks like updating each ball's position and speed, and I learned how to use things like millis()
to control the timing of events, like spawning new balls, and how to use simple math to simulate gravity's effect on the balls.
1// I'm using gravity to help tell the time. It is a constant variable that all other things can be measured against
2
3let gravity = 0.5 // Setting my value of gravity
4let ballRadius = 10
5let balls = [];
6let maxBalls = 200 // Setting the maximun number of balls to be 200
7let spawnInterval = 1000 // I want balls to
8drop in quick succession. As soon as a ball
9hits the ground another one will drop.
10let lastSpawnTime = 0
11
12function setup() {
13 createCanvas(400, 400)
14 frameRate(60)
15}
16
17function draw() {
18 background(220)
19
20 // This will draw in the existing balls
21 for (let i = 0; i < balls.length; i++) {
22 let ball = balls[i];
23 ball.y += ball.speed;
24 ball.speed += gravity;
25
26 if (ball.y + ballRadius >= height) {
27 ball.y = height - ballRadius;
28 ball.speed *= -0.8; // Add some bounce
29 }
30
31 fill(ball.color); // Fillling in random colors of each ball
32 ellipse(ball.x, ball.y, ballRadius * 2, ballRadius * 2)
33 noStroke()
34 }
35
36
37 if (balls.length < maxBalls && millis() - lastSpawnTime >= spawnInterval) {
38 balls.push({
39 x: random(width),
40 y: 0,
41 speed: 0,
42 color: color(random(255), random(255), random(255)) // Assign a random color
43 })
44 lastSpawnTime = millis();
45 }
46}
47